Developing Custom Alerts and Notifications in Trading Platforms with JavaScript
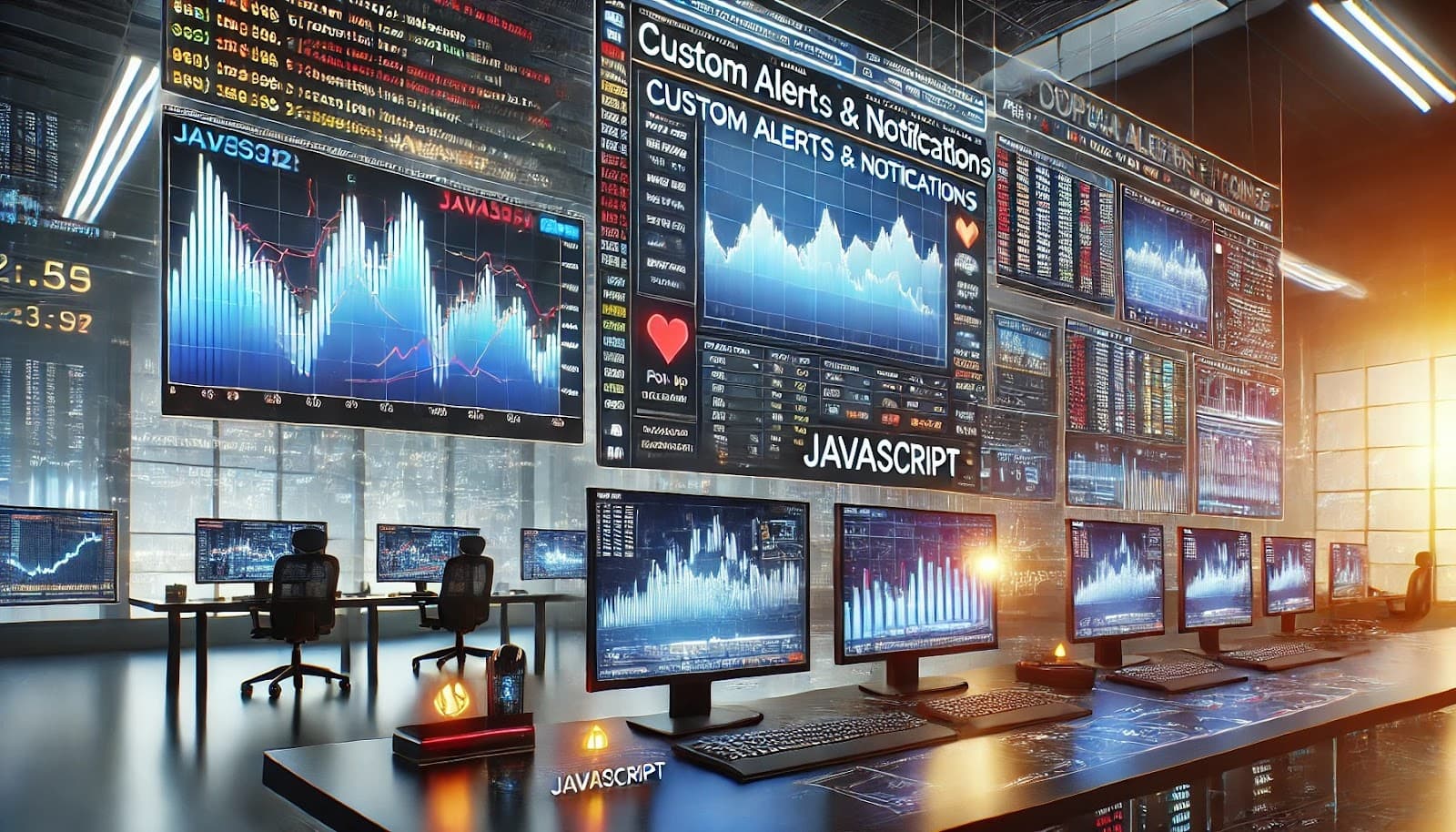
In trading platforms, custom alerts and notifications are essential tools for traders to stay informed about critical market movements and manage their positions effectively. Developing these alerts with JavaScript allows for real-time tracking and immediate notifications based on specific conditions, such as price thresholds, volume changes, or market events. JavaScript, particularly when combined with WebSockets or APIs, enables seamless integration of live data streams, making it an ideal choice for building a dynamic and responsive alert system. By setting custom triggers, traders can receive notifications through various channels, including in-app notifications, emails, or even SMS, ensuring they never miss important opportunities or risks.
An excellent example of a trading platform offering mobile alerts is the Exness mobile app, which allows users to set custom notifications for various trading conditions. Through JavaScript-powered features, developers can integrate real-time price updates and instant alerts into such apps, enabling traders to receive notifications immediately when a market reaches their set price levels. This enhances the overall trading experience by providing a user-friendly interface and timely information, allowing traders to make faster and more informed decisions while on the go. With JavaScript’s versatility and scalability, integrating custom alerts into trading platforms has never been more accessible or efficient.
What is JavaScript and How Work with Custom Alerts and Notifications
JavaScript is a high-level, dynamic programming language primarily used to create interactive effects and dynamic content on websites. It is one of the core technologies of web development, alongside HTML and CSS, enabling developers to build complex, interactive web pages and applications. JavaScript allows for things like form validation, interactive maps, and real-time updates without the need to refresh the page.
Originally developed by Netscape in the mid-1990s, JavaScript is now supported by all modern web browsers, making it an essential tool for web development. It can be used on both the client-side (in the browser) and server-side (with frameworks like Node.js), allowing developers to write full-stack applications. JavaScript is known for its flexibility, asynchronous processing, and vast ecosystem of libraries and frameworks (like React, Angular, and Vue) that streamline development.
JavaScript is highly effective in managing custom alerts and notifications on web pages, offering flexibility to interact with user actions and real-time events. Here’s how JavaScript works with custom alerts and notifications:
1. Triggering Events for Alerts
JavaScript can trigger custom alerts in response to various events, such as a user action (click, hover, etc.), or based on data updates (such as price changes or market movements). For instance, when a trader sets a price threshold, JavaScript can monitor the price continuously and show an alert when the price reaches or crosses the threshold.
Example:
let price = 100;
let alertThreshold = 120;
// Simulating price updates
function checkPrice() {
if (price >= alertThreshold) {
alert(‘Price has reached your threshold!’);
}
}
setInterval(checkPrice, 1000); // Check price every second
In this example, the checkPrice function is checking every second to see if the price has reached or exceeded the threshold and, if so, triggers an alert.
2. Displaying Alerts in the Browser
JavaScript has built-in functions like alert(), confirm(), and prompt() to display simple pop-up messages. While alert() is often used for basic notifications, it may not be ideal for complex or user-friendly notifications, especially for trading platforms.
For a more customized experience, JavaScript can dynamically create HTML elements to display custom alerts. These alerts can be styled with CSS and can include advanced features such as close buttons, icons, or animations.
Example of creating a custom alert:
function showCustomAlert(message) {
let alertDiv = document.createElement(‘div’);
alertDiv.style.position = ‘fixed’;
alertDiv.style.top = ’10px’;
alertDiv.style.right = ’10px’;
alertDiv.style.padding = ’10px’;
alertDiv.style.backgroundColor = ‘red’;
alertDiv.style.color = ‘white’;
alertDiv.innerHTML = message;
document.body.appendChild(alertDiv);
setTimeout(() => {
alertDiv.style.display = ‘none’;
}, 5000); // Hide after 5 seconds
}
showCustomAlert(‘Price alert: Threshold reached!’);
This creates a custom alert that appears in the top-right corner of the page and disappears after 5 seconds.
3. Using Notifications API for Browser-based Alerts
For more advanced and native-looking alerts, JavaScript can utilize the Notifications API to send desktop notifications directly to the user’s browser, even if they are not actively viewing the webpage. This is particularly useful for trading platforms, where users want to receive notifications about price movements, market news, or other important events without needing to keep the platform open.
To send a notification, you first need to request permission from the user:
if (Notification.permission !== “granted”) {
Notification.requestPermission();
}
// Triggering a notification
function sendNotification(message) {
if (Notification.permission === “granted”) {
new Notification(message);
}
}
// Example of sending a notification
sendNotification(‘Price alert: Threshold reached!’);
This code requests permission to send notifications if the user hasn’t granted it yet. When the permission is granted, a notification appears on the user’s desktop or mobile device.
4. Handling Multiple Alerts and Notifications
In real-time trading applications, it’s common for users to set multiple alert conditions. JavaScript can manage and track multiple alerts by storing them in arrays or objects. When a condition is met, it can loop through the stored alerts and send notifications accordingly.
Example:
let alerts = [
{ price: 120, message: ‘Price reached $120!’ },
{ price: 150, message: ‘Price reached $150!’ }
];
let currentPrice = 130;
function checkAlerts() {
alerts.forEach(alert => {
if (currentPrice >= alert.price) {
sendNotification(alert.message);
}
});
}
checkAlerts();
In this example, the checkAlerts function loops through all alerts and sends a notification if the current price meets or exceeds any of the specified thresholds.
5. Integration with Real-Time Data
JavaScript, in combination with WebSockets or APIs, can be used to monitor real-time data and trigger alerts based on live updates. For instance, a JavaScript app might use a WebSocket connection to receive live market prices. When the price hits a user-defined threshold, JavaScript can automatically trigger an alert.
Example with WebSocket:
let socket = new WebSocket(‘wss://example.com/market-data’);
socket.onmessage = function(event) {
let data = JSON.parse(event.data);
let price = data.price;
if (price >= 120) {
sendNotification(‘Price has reached your threshold of $120!’);
}
};
Here, the WebSocket connection listens for real-time market data. When the price reaches the threshold, a notification is triggered.
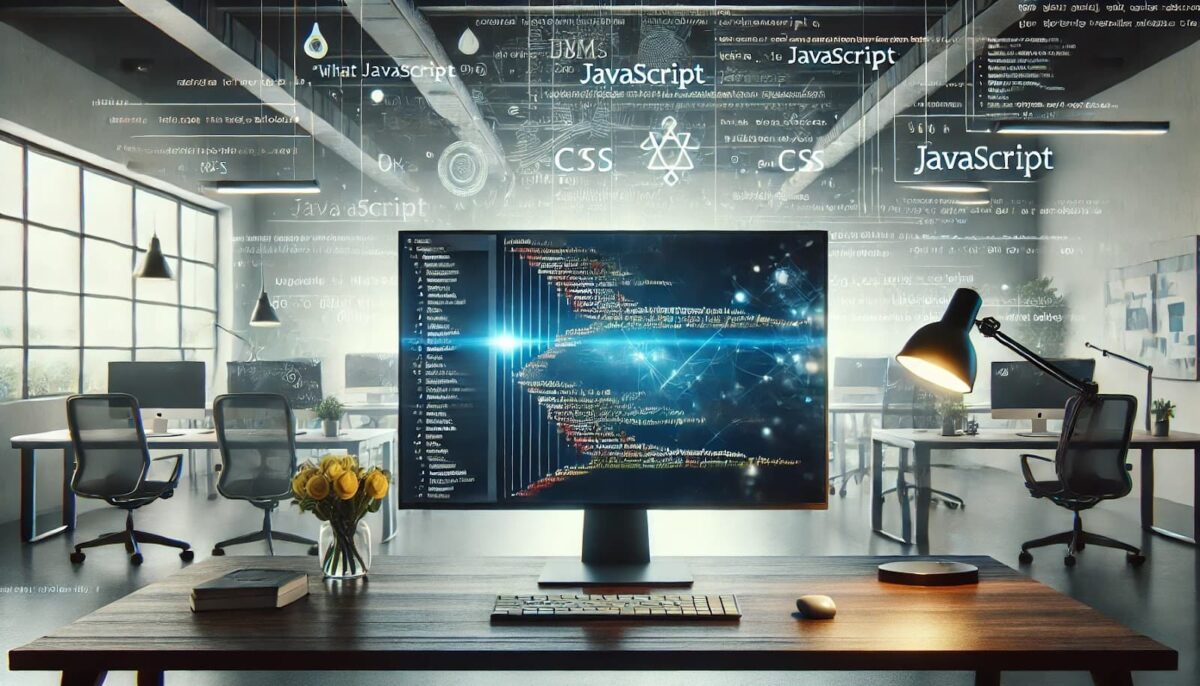
Why JavaScript is Suitable for Building Alerts
JavaScript is particularly well-suited for building alerts in trading platforms due to its ability to handle real-time, dynamic interactions on the web. One of the key features that makes JavaScript an ideal choice is its asynchronous nature, which allows it to handle multiple tasks simultaneously without interrupting the user experience. This is especially important for real-time alerts in trading, where the platform needs to continuously track and respond to market data updates without causing delays or performance issues. JavaScript can execute tasks like fetching live data, evaluating conditions, and triggering notifications all in the background, ensuring that the platform remains responsive and efficient.
Another reason JavaScript is suitable for building alerts is its broad support for various web technologies, such as WebSockets and APIs, which are often used to provide live data streams. Through WebSockets, JavaScript can establish a persistent connection to a server, receiving real-time updates on market changes like price fluctuations. This allows alerts to be triggered instantly, keeping traders informed about critical events as they happen. Additionally, JavaScript’s ability to integrate with various notification systems, such as browser pop-ups, emails, or mobile push notifications, enables developers to create customized, multi-channel alert systems. This flexibility makes JavaScript a powerful tool for enhancing trading platforms with timely and personalized alerts.
Understanding Alerts and Notifications in Trading Platforms
In trading platforms, alerts and notifications are essential tools that keep traders informed about key market events and help them manage their trades effectively. Alerts are typically set based on specific criteria or triggers, such as price movements, technical indicators, or market news. For example, a trader might set an alert to be notified when a particular stock price reaches a certain threshold, or when a moving average crossover occurs. These notifications serve as a prompt to take action, whether it’s executing a trade, analyzing the market further, or adjusting a position.
The primary goal of alerts and notifications is to enhance the trading experience by providing real-time updates without requiring traders to constantly monitor the markets. They can come in various forms, such as pop-up messages on the trading platform, email notifications, push notifications on mobile apps, or even SMS alerts. These notifications can be customized in terms of frequency, type, and delivery method, allowing traders to tailor the experience to their preferences. Effective alerts can help traders make timely decisions, minimize risks, and take advantage of market opportunities as they arise.
Best Practices for Building Trading Alerts
When building trading alerts, it’s crucial to follow best practices to ensure they are effective, reliable, and user-friendly. Here are some key best practices to consider:
- Optimize for Performance: Trading platforms handle large amounts of real-time data, so it’s essential that your alert system is optimized for performance. Avoid excessive polling of APIs, as this can cause delays or overload the server. Instead, use technologies like WebSockets for real-time updates, ensuring that alerts are triggered promptly without unnecessary lag. Additionally, minimize the impact of alerts on the platform’s overall performance by managing resources efficiently.
- Personalize Alerts for the User: Customize the alerts based on the individual user’s preferences. Allow users to set their own conditions, such as specific price thresholds or changes in technical indicators. Providing options for users to adjust the frequency, types (e.g., price alerts, volume alerts), and delivery channels (email, SMS, push notifications) will make the alert system more relevant and valuable. This personalized approach will improve the overall user experience and make alerts more actionable.
- Ensure Reliability and Accuracy: Trading decisions often rely on the accuracy of alerts, so it’s essential to build a reliable system that triggers only when the specified conditions are met. To reduce the risk of false positives, test the alert system rigorously to ensure that it is precise and consistent. Implement proper error handling and fail-safes to prevent missed alerts or inaccurate notifications that could negatively impact trading decisions.
- Keep Alerts Clear and Concise: The purpose of trading alerts is to provide timely and relevant information. Ensure that the content of each alert is clear, concise, and actionable. Avoid overloading users with unnecessary details, and instead focus on the key information that will help traders make informed decisions. For example, a price alert should include the asset name, the triggered price level, and an indication of the direction of the price change.
- Scalability and Flexibility: As your platform grows, so will the number of users and the volume of alerts generated. Design the alert system with scalability in mind, allowing it to handle an increasing load without compromising performance. Consider using cloud services and distributed systems to support the growing demand. Additionally, ensure that the system is flexible enough to accommodate future feature enhancements, such as the integration of new types of alerts or external services.
Conclusion
Developing custom alerts and notifications in trading platforms using JavaScript offers significant advantages in enhancing user experience and improving decision-making. JavaScript’s asynchronous capabilities, along with its ability to interact with real-time data through WebSockets and APIs, enable seamless, real-time alerts that are critical for traders. By using JavaScript, developers can create flexible, responsive, and personalized notification systems that notify users about important market events, such as price movements or trading opportunities, in a timely manner.
Recent Comments