Mastering JavaScript Basics – A Comprehensive Guide
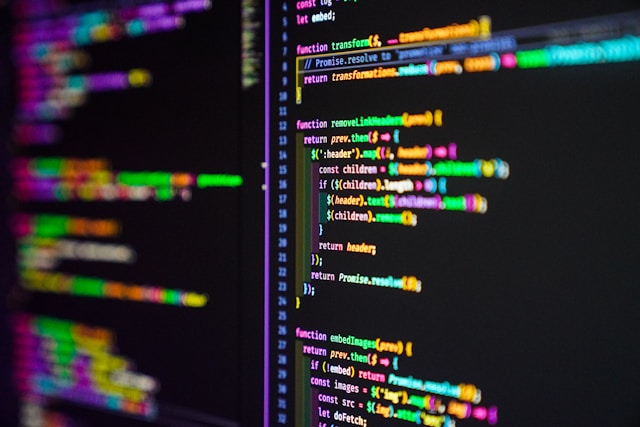
JavaScript stands as a robust and adaptable programming language, much like the diverse gaming experiences available at aviatorkz.kz, capable of infusing your website with interactivity and dynamic features. In this detailed guide, we’ll dive into the core principles of JavaScript and uncover its vast potential. By the conclusion of this piece, you’ll possess a solid understanding of JavaScript’s fundamentals, equipping you with the skills to develop interactive and captivating web applications.
Introduction to JavaScript
JavaScript, often referred to as JS, was invented by Brendan Eich and has since become one of the most popular web technologies. It allows you to enhance your website by adding features like responsive buttons, dynamic styling, animations, and much more. JavaScript is both versatile and beginner-friendly, making it accessible to developers of all levels.
The Power of JavaScript
JavaScript’s strength lies in its flexibility and extensibility. Developers have built numerous tools and APIs on top of the core language, expanding its functionality with minimal effort. Here are some key components that unlock JavaScript’s potential:
Browser Application Programming Interfaces (APIs)
These built-in web browser APIs enable dynamic HTML creation, CSS styling, access to a user’s webcam, and even 3D graphics and audio manipulation. JavaScript seamlessly integrates with these APIs to create interactive web experiences.
Third-party APIs
Developers can leverage third-party APIs from platforms like Twitter and Facebook to incorporate external functionality into their websites. This allows for the integration of social media feeds, authentication systems, and more.
Frameworks and Libraries
JavaScript offers a vast ecosystem of frameworks and libraries that expedite web development. These tools simplify tasks such as DOM manipulation, data handling, and UI design, making web development more efficient.
Getting Started with JavaScript
Creating a “Hello World!” Example
Let’s kickstart your JavaScript journey with a classic “Hello World!” example. To begin, follow these steps:
- If you haven’t done so already, create a folder named “scripts” in your project directory.
- Inside the “scripts” folder, create a new text document named “main.js” and save it.
- Open your “index.html” file and insert the following code just before the closing </body> tag:
htmlCopy code
<scriptsrc=”scripts/main.js”></script>
This script tag imports your JavaScript code into the HTML file, allowing it to interact with the page’s content.
- In your “main.js” file, add the following JavaScript code:
const myHeading = document.querySelector(“h1”); myHeading.textContent = “Hello world!”;
This code selects the <h1> element on your page and changes its content to “Hello world!” using JavaScript.
- Save both the HTML and JavaScript files.
- Load your “index.html” file in your browser, and you should see the heading text changed to “Hello world!” thanks to JavaScript.
Understanding JavaScript Basics
To grasp the fundamentals of JavaScript, let’s explore some core concepts:
Variables
Variables are containers that store values. In JavaScript, you can declare a variable using the let keyword. For example:
let myVariable = “Hello, World!”;
You can change the value of a variable after it’s declared:
myVariable = “New value!”;
JavaScript supports various data types, including strings, numbers, booleans, arrays, and objects.
Comments
Comments are essential for code readability. You can add comments in JavaScript using /* */ for multiline comments or // for single-line comments:
/* This is a multiline comment. It spans multiple lines. */// This is a single-line comment.
Operators
Operators perform operations on variables and values. JavaScript includes operators for addition, subtraction, multiplication, division, assignment, and comparison:
let sum = 5 + 3; let difference = 10 – 4; let product = 2 * 6; let quotient = 8 / 2; let isEqual = 5 === 5; // truelet isNotEqual = 3 !== 2; // true
Conditionals
Conditionals are used to make decisions in your code. The if…else statement is commonly used to execute different code blocks based on a condition:
let temperature = 25; if (temperature > 30) { console.log(“It’s hot outside!”); } else { console.log(“It’s a pleasant day.”); }
Functions
Functions allow you to package and reuse code. You can define your functions like this:
functionmultiply(num1, num2) { let result = num1 * num2; return result; }
You can then call the function and pass arguments:
let product = multiply(4, 5); // product is 20
Events
JavaScript can respond to user interactions through event handling. For example, you can add an event listener to execute code when a button is clicked:
document.querySelector(“button”).addEventListener(“click”, function() { alert(“Button clicked!”); });
Conclusion
This comprehensive guide has provided you with a solid foundation in JavaScript basics. You’ve learned about variables, comments, operators, conditionals, functions, and event handling. As you continue to explore JavaScript, remember that practice and experimentation are key to becoming proficient in this versatile programming language.
Start incorporating JavaScript into your web projects and watch your websites come to life with interactivity and dynamic functionality. Whether you’re a beginner or an experienced developer, JavaScript offers endless possibilities for enhancing your web development skills.
Recent Comments